외부에서 자료를 입력받아 실시간으로 그래프를 업데이트 하여 출력하는 그래프를 만들어보고자 한다. 매번 만들다가 코드 어디에다 정리해 놨지 잃어버리고... 이번에 확실히 정리해 두자.
1. 간단한 그래프 그리기
import matplotlib.pyplot as plt
import numpy as np
from matplotlib.animation import FuncAnimation
#fig, ax = plt.subplots()
fig = plt.figure()
ax = plt.axes(xlim=(0, 3), ylim=(0, 9))
a = np.arange(0, 3, 0.2)
ax.plot(a, a**2)
plt.show()
- subplots을 이용하면 객체지향으로 작성 가능, figure(), axes()를 이용해 개별 호출 가능
- figure는 영어로 "모양, 도형" 이라는 뜻인데 그래프가 그려질 "도화지" 라고 생각해 보자.
- axes는 영어로 "축" 이라는 뜻인데 축을 지정함으로써 "그래프를 정의"하는 것으로 생각해 보자.
- 이 그래프는 x^(2)의 식을 그린다.
<실행 결과>
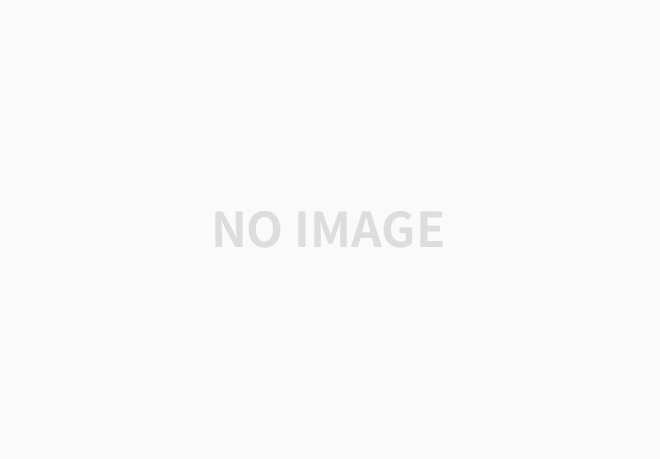
2. 움직이는 그래프 그리기
이차함수 위에서 움직이는 점을 그릴 것이다.
(reference : https://riptutorial.com/matplotlib/example/23558/basic-animation-with-funcanimation)
import matplotlib.pyplot as plt
import numpy as np
from matplotlib.animation import FuncAnimation
fig = plt.figure()
ax = plt.axes(xlim=(0, 3), ylim=(0, 9))
a = np.arange(0, 3, 0.2)
ax.plot(a, a**2)
redDot, = plt.plot([], [], 'ro')
def animate(frame):
redDot.set_data(frame, frame**2)
return redDot
ani = FuncAnimation(fig, animate, frames=np.array([0.2, 0.4, 0.6]))
plt.show()
<실행결과>
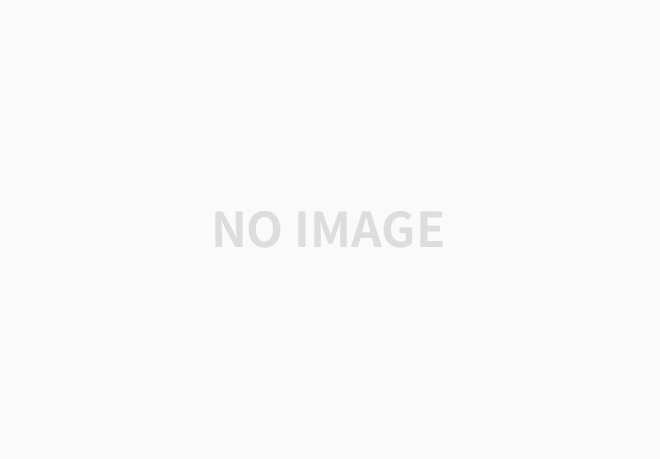
움직이는 것을 볼 수 있을텐데 캡쳐는 image로만 저장이 되어 아쉽다.
gif로 저장하기 위해선 imagemagick을 설치해야 하는데 귀찮다. 동영상으로 먼저 저장해야겠다.
3. 동영상으로 저장하기
(reference : https://stackoverflow.com/questions/37146420/saving-matplotlib-animation-as-mp4)
import matplotlib.pyplot as plt
import numpy as np
from matplotlib.animation import FuncAnimation
import matplotlib.animation as animation
fig = plt.figure()
ax = plt.axes(xlim=(0, 3), ylim=(0, 9))
a = np.arange(0, 3, 0.2)
ax.plot(a, a**2)
redDot, = plt.plot([], [], 'ro')
def animate(frame):
redDot.set_data(frame, frame**2)
return redDot
ani = FuncAnimation(fig, animate, frames=np.array([0.2, 0.4, 0.6]))
FFwriter = animation.FFMpegWriter(fps=1)
ani.save('animation.mp4', writer = FFwriter)
<실행결과>
fps를 작게 하지 않으면 순식간에 지나간다.
동영상을 gif로 저장해도 될 듯. (난 추가 라이브러리 설치가 매우매우 싫다)
4. 움직인 자취 남기기
움직인 자취를 남긴다면 추척하면서 보기에 더 좋을 것 같다.
import matplotlib.pyplot as plt
import numpy as np
from matplotlib.animation import FuncAnimation
import matplotlib.animation as animation
fig = plt.figure()
ax = plt.axes(xlim=(0, 3), ylim=(0, 9))
a = np.arange(0, 3, 0.2)
ax.plot(a, a**2)
x, y = [], []
redDot, = plt.plot([], [], 'ro')
def animate(frame):
x.append(frame)
y.append(frame**2)
redDot.set_data(x, y)
return redDot
ani = FuncAnimation(fig, animate, frames=np.array([0.2, 0.4, 0.6]))
FFwriter = animation.FFMpegWriter(fps=1)
ani.save('animation.mp4', writer = FFwriter)
<실행화면>